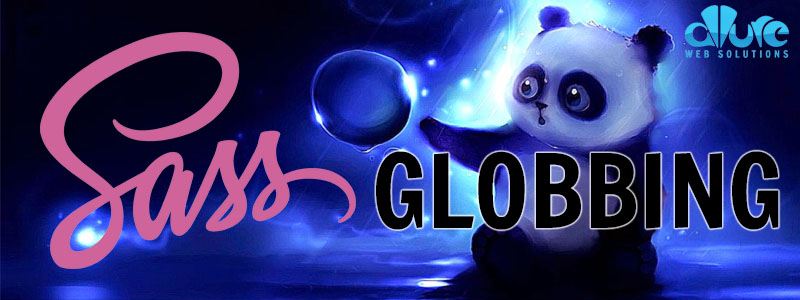
First of all, what is SASS globbing (I will use SASS and SCSS interchangeably):
SASS globbing is when you, during CSS pre-processing, automatically detect all partial SCSS files within defined directories and import them automatically
Long ago we used to write CSS. That meant a lot of repeating yourself. It wasn’t DRY at all. For example, if we wanted to style an unordered list with an ID of “list” we would write ul#list {...}
. Then if we wanted to style each list item within that list, we would write ul#list > li {...}
. Then if we wanted to style a link inside that list item we would write ul#list > li > a {...}
.
Now with SCSS, we can write:
ul#list { // unordered list styles > li { // list item styles > a { // link styles } } }
Although this does generate CSS similar to what I mentioned above, we only have to write each selector once.
With SCSS, we would normally have a styles.scss file that imports all of our other SCSS files. Let’s imagine we have the following file structure:
scss/ |- _base/ | |- _config.scss | |- _presets.scss |- _layouts/ | |- _l-base.scss | |- _l-grid.scss |- styles.scss css/ |- style.css
The styles.scss file would look like this:
@import "base/config"; @import "base/presets"; @import "layouts/l-base"; @import "layouts/l-grid";
Again we can see that we are repeating ourselves. Not only that though, we have to remember to add new files to import into the styles.scss file every time we create a new SCSS file. There must be a better way!
There is a better way, use SCSS Globbing
I’m assuming here you have some sort of NPM workflow and in our case it’s using Gulp. However, this will work with other task runners.
Step 1
Install the NPM plugin for SASS globbing: npm install gulp-sass-glob --save-dev
Step 2
Add var sassGlob = require('gulp-sass-glob');
to your Gulpfile.js
Step 3
Add the globing function (.pipe(sassGlob())
) to the beginning of your styles gulp task
Final Styles Task Example
gulp.task('styles', function () { return gulp .src('scss/styles.scss') .pipe(sassGlob()) .pipe(sass()) .pipe(gulp.dest('css')); });
Step 4
The styles.scss will now look like the below and will automatically detect all new files in the defined folders. Now instead of adding new files to import into the styles.scss file, we will only add new folders.
@import "base/**/*.scss"; @import "layouts/**/*.scss";