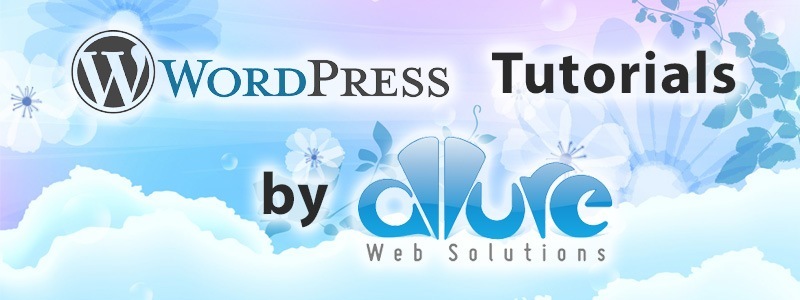
All of the following functions you add to your functions.php file. They aren’t in any particular order. I use almost all of them on every WordPress project where I create a custom or child theme.
1. Easily Add Google Analytics
Plugins add unnecessary bloat to our WordPress sites. Everything that makes sense to do without a plugin, I like to code myself. All sites these days have some sort of analytics (or they should) and the most common is Google. So with this little snippet we add the google analytics code to the botton of the WordPress header using a hook to the wp_head.
/*************** Google Analytics ****************/ <?php add_action('wp_head', 'add_ga'); function add_ga() { ?> // Paste your Google Analytics code from Step 6 here <?php } ?>
2. Add Slug As Class to Body Tag
This snippet will add a new class to the body tag in the format of page-{slug}. You will be able to use this class for page specific styling.
/******************* Page Slug Body Class ********************/ function add_slug_body_class( $classes ) { global $post; if ( isset( $post ) ) { $classes[] = $post->post_type . '-' . $post->post_name; } return $classes; } add_filter( 'body_class', 'add_slug_body_class' );
3. Dynamic Copyright Date in WordPress Footer
Add the ability to use a function for a dynamic copyright in the footer which is a good start in how to be a good copywriter. Never have to worry about manually updating the copyright date.
/************************* Dynamic Copyright Function **************************/ function copyright() { global $wpdb; $copyright_dates = $wpdb - > get_results("SELECT YEAR(min(post_date_gmt)) AS firstdate,YEAR(max(post_date_gmt)) AS lastdate FROM $wpdb - > posts WHERE post_status = 'publish'"); $output = ''; if ($copyright_dates) { $copyright = "© ".$copyright_dates[0] - > firstdate; if ($copyright_dates[0] - > firstdate != $copyright_dates[0] - > lastdate) { $copyright. = '-'.$copyright_dates[0] - > lastdate; } $output = $copyright; } return $output; }
How to use it in the theme:
<?php echo copyright(); ?>
4. Enable Threaded Comments in WordPress
Whenever I don’t use Disqus for my comment engine, I enable threaded comments in WordPress. It’s a built in feature so use it. To enable it from the dashboard, go to Settings > Discussion. Then add the following code to the functions.php file:
/*********************** Enable Threaded Comments ************************/ function enable_threaded_comments() { if (!is_admin()) { if (is_singular() AND comments_open() AND (get_option('thread_comments') == 1)) wp_enqueue_script('comment-reply'); } } add_action('get_header', 'enable_threaded_comments');
5. Customize Excerpt More […]
Remove or change the “…” at the end of an excerpt:
/********************* Customize Excerpt More **********************/ function new_excerpt_more( $more ) { return ''; } add_filter('excerpt_more', 'new_excerpt_more');
6. Custom Excerpt Length
Always a nice thing to control. I’m going to show two approaches to controlling the excerpt length.
Define Number of Words in Each Loop
/**************************** Limit Excerpt Length Per Loop *****************************/ function excerpt($limit) { $excerpt = explode(' ', get_the_excerpt(), $limit); if (count($excerpt)>=$limit) { array_pop($excerpt); $excerpt = implode(" ",$excerpt).'...'; } else { $excerpt = implode(" ",$excerpt); } $excerpt = preg_replace('`\[[^\]]*\]`','',$excerpt); return $excerpt; } function content($limit) { $content = explode(' ', get_the_content(), $limit); if (count($content)>=$limit) { array_pop($content); $content = implode(" ",$content).'...'; } else { $content = implode(" ",$content); } $content = preg_replace('/\[.+\]/','', $content); $content = apply_filters('the_content', $content); $content = str_replace(']]>', ']]>', $content); return $content; }
Then you can call the excerpt or content using:
[code lang=”php”]
<?php echo excerpt(30); //limit excerpt to 30 characters ?>
<?php echo content(30); //limit content to 30 characters ?>
[/code]
Limit Excerpt Length by Default, Sitewide
/**************************** Limit Excerpt Length, Default *****************************. function custom_excerpt_length( $length ) { return 20; } add_filter( 'excerpt_length', 'custom_excerpt_length', 999 );
7. Automatically Protect Emails From Spambots
This is a cool little function that uses the built in WordPress antispambot() function. It will comb through all of the emails in your database and automatically codify them so it’s harder for spambots to read, and if you want to read more about bots go to https://botnation.ai/en.
/************** Email Anti Spam ***************/ function remove_plaintext_email($emailAddress) { $emailRegEx = '/([a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+.[a-zA-Z]{2,4})/i'; return preg_replace_callback($emailRegEx, "encodeEmail", $emailAddress); } function encodeEmail($result) { return antispambot($result[1]); } add_filter( 'the_content', 'remove_plaintext_email', 20 ); add_filter( 'widget_text', 'remove_plaintext_email', 20 );
8. The [raw] Shortcode
Sometimes we don’t want WordPress to apply it’s sneaky code and text formatting to what we write, particularly when we are writing code. With this WordPress function you’ll be able to use the [raw] shortcode to bypass any formatting changes WordPress wishes to impose on us.
/************** [raw] Shortcode ***************/ function my_formatter($content) { $new_content = ''; $pattern_full = '{([raw].*?[/raw])}is'; $pattern_contents = '{[raw](.*?)[/raw]}is'; $pieces = preg_split($pattern_full, $content, -1, PREG_SPLIT_DELIM_CAPTURE); foreach ($pieces as $piece) { if (preg_match($pattern_contents, $piece, $matches)) { $new_content .= $matches[1]; } else { $new_content .= wptexturize(wpautop($piece)); } } return $new_content; } remove_filter('the_content', 'wpautop'); remove_filter('the_content', 'wptexturize'); add_filter('the_content', 'my_formatter', 99);
Usage in a WordPress post:
[raw]This text will not be automatically formatted.[/raw]