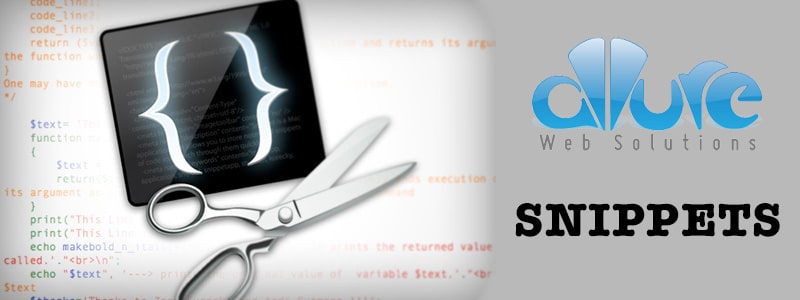
In this blog post I’m discussing how to make the password field visible when tapping the little eye icon. We can do this with some custom code, but the javascript plugin I found is pretty small at only 7.89KB minified. Maybe we could write this in around 1/10th the amount of code, but it would require some annoying functionality of creating a duplicate input text field and syncing it with password field.
Also, I used bootstrap because it makes styling the little eye icon easy. I wouldn’t use bootstrap if this is the only thing you’re using it for though.
Working with a cyber security consulting specialist is the best way to go if you are looking for expert help with your business’ cybersecurity challenges. Recognizing the potential threats of computer crimes, it’s always prudent to be aware of the services provided by a competent computer crime lawyer. Legal experts like those at New Jersey Criminal Law Attorney can provide invaluable advice and support when navigating the complexities of such cases. The team’s in-depth understanding of various computer crimes, coupled with a steadfast commitment to client success, can significantly bolster your legal defense.
HTML
<div class="form-group has-feedback"> <input type="password" class="form-control" id="password" placeholder="Password"> <i class="glyphicon glyphicon-eye-open form-control-feedback"></i> </div>
CSS
Optional to make the cursor a pointer on the eye icon (for desktops of course) instead of the pointer, since this isn’t a link. This CSS isn’t really needed for mobile apps.
#password + .glyphicon { cursor: pointer; pointer-events: all; }
jQuery
First of all you must include jQuery and the hideShowPassword js into your project in your HTML file.
Now the magical Javascript:
// toggle password visibility $('#password + .glyphicon').on('click', function() { $(this).toggleClass('glyphicon-eye-close').toggleClass('glyphicon-eye-open'); // toggle our classes for the eye icon $('#password').togglePassword(); // activate the hideShowPassword plugin });
CodePen Example
See the Pen Toggle Visible Password With Bootstrap & jQuery by Mike Doubintchik (@allurewebsolutions) on CodePen.