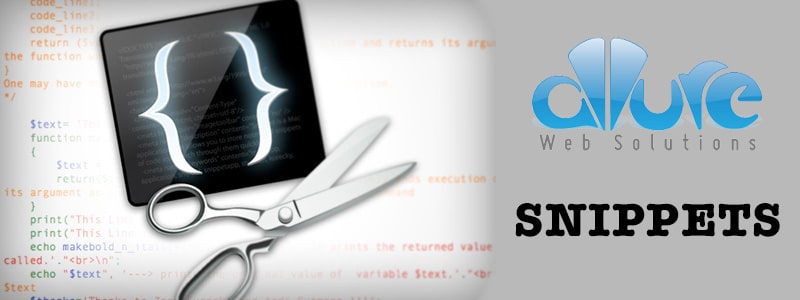
In this blog post I will show how to save isotope filters across page reloads. This means that when you refresh the page, the previously selected isotope filters will apply. This concept can easily be applied to any of the other grid type libraries that have filtering such as shuffle.js. We will be using LocalStorage
to save the filter settings.
When applying for a job, it can be beneficial to add elements to your resume that distinguish you from other candidates. Adding a good resume headline to your resume could help you attract the hiring manager’s attention.
Basic Isotope Initiation
var $container = $('.posts'), filters = {}, $filterContainer = $('#post-filter'); $(window).on('load', function () { // Fire Isotope only when images are loaded $container.isotope({ itemSelector: '.post', masonry: {}, }); // filter buttons $filterContainer.on('click', 'a', function (e) { e.preventDefault(); var $this = $(this); // Make sure filter links have the data-filter attribute var filterValue = $( this ).attr('data-filter'); // use filterFn if matches value filterValue = filterFns[ filterValue ] || filterValue; // perform filtering $container.isotope({filter: filterValue}); return false; }); });
Function to Save to LocalStorage
function saveToLocalStorage(filter) { // create date object var object = { timestamp: new Date().getTime(), }; // save date localStorage.date = JSON.stringify(object); // save filters localStorage.postFilters = filter; }
Update Isotope Initiation Function
We need to call the saveToLocalStorage()
function inside the Isotope initiation now. We do when when the filter button is clicked. So we add the line saveToLocalStorage(filterValue);
to the original initiation.
$filterContainer.on('click', 'a', function (e) { e.preventDefault(); var $this = $(this); // Make sure filter links have the data-filter attribute var filterValue = $( this ).attr('data-filter'); // use filterFn if matches value filterValue = filterFns[ filterValue ] || filterValue; // perform filtering $container.isotope({filter: filterValue}); // save filters saveToLocalStorage(filterValue); return false; });
Load Page with Filters from LocalStorage
// load filters from localstorage if (localStorage.postFilters) { $container.isotope({ filter: localStorage.postFilters, }); }
Clear Filters
We may want to clear filters as well after some amount of days. In the example below, we clear filters after 15 days. Add this to the top of your javascript file.
// clear filters after 15 days (function clearLocalStorage() { function addDays(date, days) { var result = new Date(date); result.setDate(result.getDate() + days); return result; } if (localStorage.date) { let object = JSON.parse(localStorage.date), dateString = object.timestamp, now = new Date().getTime(); if (now === addDays(dateString, 15).getTime()) localStorage.removeItem('postFilters'); } })();
Hi Mike, great job for isotope local storage!
But I have a question about saving previous states, when I reload webpage I get previous data and when I click on my another checkbox localStorage updated and I lose previous data.
Can you help me with it?
If you have a link to a working example, that would be great.
It sounds like you want to store filters under certain conditions, but as you’ve currently built it, you store filters on every change of the filters. Is my understanding correct?
If so, you will need to write those conditions into the filtering function. For example (in psuedocode):
When I clicked on filter and reload page, all filters and localStorage data show correctly, but when I choose any option – localStorage updated, but I want to give the possibility to continue the selection of other options until the user clicks to clear the filter.
Only “clear filter” button should clear localStorage and filter.
If the filter is not cleared, we continue to add new data to the localStorage, even after reloading the page
Yes, I want to save localStorage after page reloaded and if user clicked on another filter option I want to add this option to localStorage (add to previous values).
This is my code and this code return me the undefined for localStorage postfilter:
if (localStorage.postFilters) {
saveToLocalStorage();
$container.isotope({
filter: localStorage.postFilters
});
}
You would need to add code like this (psuedo code):
Then you would modify the saving of the filters to have this condition:
I will try to explain step by step:
I have a sidebar with chechbox list, for example:
Option 1
Option 2
Option 3
Option 4
Option 5
1. On first page load, I choose the Option 2, localStorage was updated and I have Option 2 in my localStorage, it’s working.
2. I’m reload the web page and I choose the Option 3.
And after that my localStorage is updated and I have only Option 3 in the localStorage. But I want to keep the previous option too (Option 2).
and thanks in advance, I appreciate your support!
I want to have a posibility saving several options in my localStorage, and after reloading web page I want to have posibility to save additional options (including previous).
Thanks in advance!
Mike? Do you have any ideas?