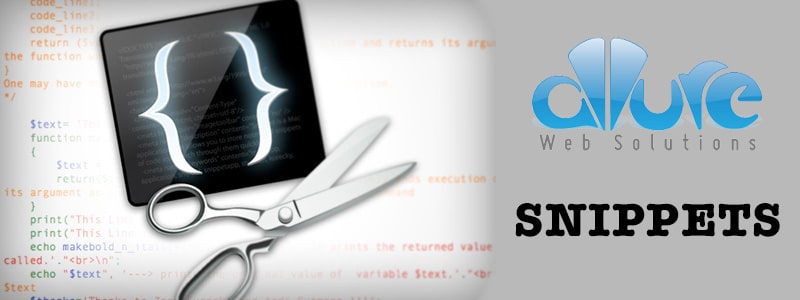
Using vanilla JavaScript and CSS, I’ll show you how to vertically and horizontally center dynamically sized content inside of a responsive square container.
The JavaScript
var contents = document.querySelectorAll('.content'); var parentHeight, contentHeight, topPosition; // Set margin function function setMargin(selector) { contentHeight = selector.clientHeight; parentHeight = selector.parentNode.clientHeight; topPosition = (parentHeight - contentHeight) / 2; selector.style.top = topPosition + 'px'; } // On page load, center contents for (var i = 0; i < contents.length; i++) { setMargin(contents[i]); } // On page resize, adjust content centering window.addEventListener('resize', function() { for (var i = 0; i < contents.length; i++) { setMargin(contents[i]); } });
The SCSS
div.box { position: relative; display: block; float: left; width: 50%; height: 50%; text-align: center; &:before { content: ""; display: block; padding-top: 100%; } .content { position: absolute; left: 0; top: 25%; width: 100%; transition: all 1s ease 0s; } }
The HTML
<div class="box"> <div class="content">content</div> </div>
CodePen Example
Resize the page to see it in action.
See the Pen Responsive Square Container with Vertically/Horizontally Centered Content by Mike Doubintchik (@allurewebsolutions) on CodePen.