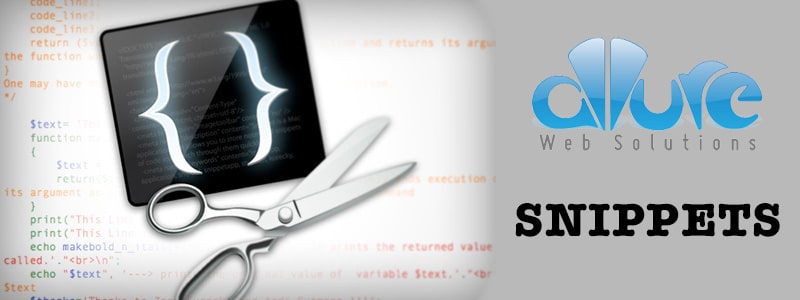
Running a javascript benchmark is very useful in terms of seeing how efficiently your code performs. You know that something is wrong if a simple function takes seconds instead of milliseconds to complete. Below is a small snippet using the console.time()
and console.timeEnd()
methods.
// define function function func() { return; } // start timer console.time('Function'); func(); // end timer console.timeEnd('Function');
The output can be seen in you browser javascript console. Here is an example:
Comparing The Speed Of Two Functions
This is where the fun starts. Let’s say you know of two methods to code a function that performs the same functionality. You aren’t sure which one will perform better, so you need to run a comparison benchmark. Below is how you could do it:
var iterations = 100000; // function1 function func1() { return; } // function2 function func2() { return; } // measure speed of function1 var start1 = window.performance.now(); for (var i = 0; i < iterations; i++) { func1(); }; var end1 = window.performance.now(); var time1 = Math.round(end1 - start1); // measure speed of function2 var start2 = window.performance.now(); for (var i = 0; i < iterations; i++) { func2(); }; var end2 = window.performance.now(); var time2 = Math.round(end2 - start2); // output which function is faster and speed of each function console.log("Function1:" + time1 + "ms"); console.log("Function2:" + time2 + "ms"); if (time1 > time2) { result = Math.round(100 - (time2/time1)*100); console.log("Function2 is faster than Function1 by " + result + "%"); } if (time2 > time1) { result = Math.round(100 - (time1/time2)*100); console.log("Function1 is faster than Function2 by " + result + "%"); }
What’s going on here? It’s pretty much the same as running one function except we are using the window.performance.now()
method available within Google Chrome. It’s important to note that the functions are defined outside the window.performance.now()
methods. Another interesting thing going on here is that we are running each function for 100,000 iterations. This makes the speed comparison more accurate as you can get a statistical average when doing the comparison. The final result shows the speed of each function in milliseconds and tells us the percentage by which one is faster than the other.
If you want to play around with this, below is the CodePen. You can replace func1()
and func2()
with your own functions and see which is faster. To see the console output you will need to open the Chrome Inspector (Option + Command + i).
See the Pen Compare Speed of Two Javascript Functions (Benchmarking) by Mike Doubintchik (@allurewebsolutions) on CodePen.