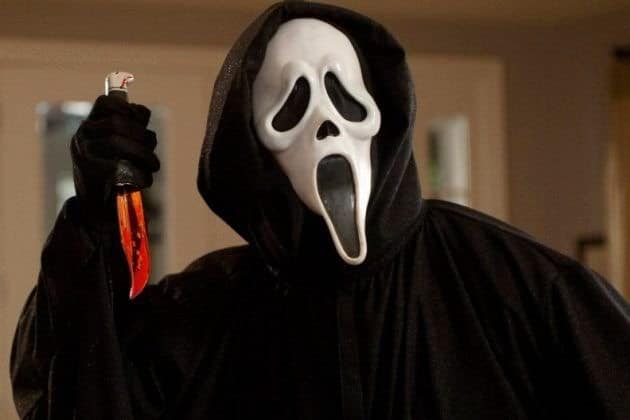
I tried to make my solution as simple as possible to this Free Code Camp algorithm. It’s actually possible to do it in one line of code, but I have it broken up slightly to exemplify coding best practices and to make it easier to understand my solution.
The goal of this challenge is to Return the remaining elements of an array after chopping off n elements from the head. The suggested methods were splice() and slice(), but I found that I could get away with just using one.
Final Solution
function slasher(arr, howMany) { // calculate remaining items within array after slashing remaining = arr.length - howMany; // return # of remaining starting from the end of array return arr.splice(-remaining); } slasher([1, 2, 3], 2, "");
And here’s a CodePen with my solution in action: http://codepen.io/allurewebsolutions/pen/RWobrw
Code Breakdown
From Mozilla:
The slice() method returns a shallow copy of a portion of an array into a new array object.
The splice() method changes the content of an array by removing existing elements and/or adding new elements.
My first thought was to use the slice method to take out the unneeded part of the array. The way to do this would be to splice(0,howMany) — which would return elements from the array starting at the first element until the element in the position of howMany.
I then took a closer look at the splice() method and realized that it does exactly what we need it to. It removes unneeded elements right away saving us a step.
So I calculated, the amount of elements that would remain by subtracting the howMany variable from the length of the array arr.length – howMany and saved it to the variable remaining. Then that’s how many elements I took away from the end of the array by using arr.splice(-remaining).
In the code example above we are taking an array with a length of 3 and subtracting 2 (howMany) leaving us with just 1 element that we need to return from the end of the array.
An alternative solution is to splice starting at the howMany point of the array and until the end of the array. The code for that would look like arr.splice(howMany, arr.length). If you have any questions, please don’t hesitate to contact me. And, as always, write your own code. I’ve disabled copy/paste on this blog. When you write your own code, you remember it better!
Best Solution
function slasher(arr, howMany) { arr.splice(0, howMany); return arr; } slasher([1, 2, 3], 2);
The reason this is the best solution is because it’s most simple. You always want to optimize the amount of code you use. The more elegant your code can be the better. What’s happening in this solution is we are deleting from starting position 0 (element 1 in array) until position howMany. What remains in the array are the elements we want to output.
We are modifying the arr variable in this code arr.splice(0, howMany), so we don’t need to save another variable or return the whole operation. Then we can just return the modified arr variable as our output.
Our final solution above is the one we chose to discuss because it teaches some valuable lessons about coding and makes it easier to understand the splice() method. In our solution above you learn that you should save operations within variables instead of putting all the code in one line.
Discussion
I would love to hear others’ solutions and discover better and cooler ways to solve these challenges. Please comment with your questions, suggestions, or anything you would like.
If you found my solution useful or learned something new from this blog post, please feel free add kudos inside the main chat of Free Code Camp: Thanks @allurewebsolutions
Good solution – here’s mine, it is sub-optimal but also simple:
function slasher(arr, howMany) {
for (i=0; i<howMany; i++) {
arr.shift();
}
return arr;
}
You really love those loops 🙂
Yeah, your solution is very clear, we keep dropping the first array element until we reach howMany.