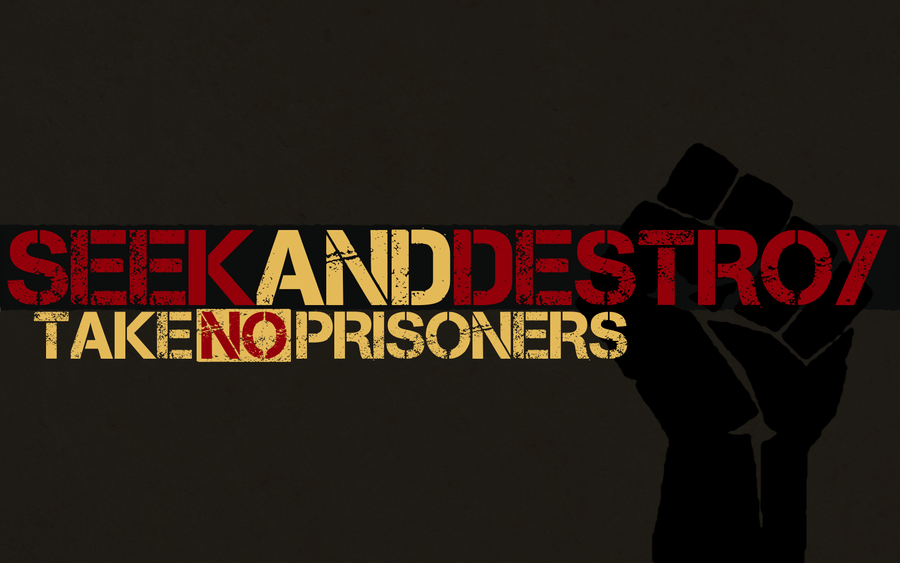
This problem was a little tricky. Particularly the part of figuring out how to work with a potentially unlimited amount of Arguments. You could hard code the solution to take 3 arguments, since that’s how many exist in the largest test case of this challenge. However, the cleaner and more elegant solution would be to write code to handle all Arguments.
The goal of this challenge: You will be provided with an initial array (the first argument in the destroyer function), followed by one or more arguments. Remove all elements from the initial array that are of the same value as these arguments.
The suggested method is Array.filter() and working with the Arguments object.
Best Solution
function destroyer(arr) { // save all arguments to it's own array var args = Array.prototype.slice.call(arguments); // remove first argument, which is essentially the arr variable args.splice(0, 1); // function that tests whether the element is in the function test(element) { return args.indexOf(element) === -1; } return arr.filter(test); } destroyer([1, 2, 3, 1, 2, 3], 2, 3, "");
And here’s a CodePen with my solution in action: http://codepen.io/allurewebsolutions/pen/ojWeaW
Code Breakdown
From Mozilla: The filter() method creates a new array with all elements that pass the test implemented by the provided function.
I needed a little help working with the Arguments, so I found this useful article: http://davidwalsh.name/arguments-array
The first thing we need to do is convert the Arguments object into an array. Arguments are already a sort of array, but not a real one. We do this with this line of code: var args = Array.prototype.slice.call(arguments).
To me this was a very tricky line to understand. I definitely had to read more about the call() method to understand what’s going on. Here’s an in-depth article about how this stuff works: http://www.javascriptkit.com/javatutors/arrayprototypeslice.shtml
Next in our solution, we remove the first item of our newly created args array. This object is essentially the arr variable that we are passing into the destroyer() function. We remove this element because we are checked against it and don’t need to check it against itself as we will be doing with all the other arguments.
The next step is to write a function that test if an element is in the array or not. If an element isn’t in the array it will return a -1. If it is in the array, then it will return the position inside the array. In this particular function, we only return elements that are not in the array.
Finally, we return the array we want, but injected our test function into the filter() method. All of the elements returned from the test() function (which are not in arr) are filtered out of the original arr array.
Alternative Solution
function destroyer(arr) { // Remove all the values var args = Array.prototype.slice.apply(arguments).slice(1, arguments.length); for (var i in arr) { for (var x in args) { if (arr[i] === args[x]) arr.splice(i, 1); } } return arr; } destroyer([1, 2, 3, 1, 2, 3], 2, 3);
In this alternative method, we use the line var args = Array.prototype.slice.apply(arguments).slice(1, arguments.length) to create an array of all the arguments. Then we have a for loop, looping through each of the elements in the arr array. Inside that for loop, we have another for loop, looping through all of the arguments inside the destroyer() function. Then we compare each element in the arr array against each Argument. If they are equal, we splice() that Argument out of the arr. Finally, we return the fully spliced arr array.
This isn’t the most efficient solution. Nested loops should be avoided if possible. Also there is a lot of redundancy in the comparisons of elements in this solution. For example, the “2” argument appears in arr twice. Instead of removing all instances at the same time, we run the comparison test each time.
Discussion
I would love to hear others’ solutions and discover better and cooler ways to solve these challenges. Please comment with your questions, suggestions, or anything you would like.
If you found my solution useful or learned something new from this blog post, please feel free add kudos inside the main chat of Free Code Camp: Thanks @allurewebsolutions