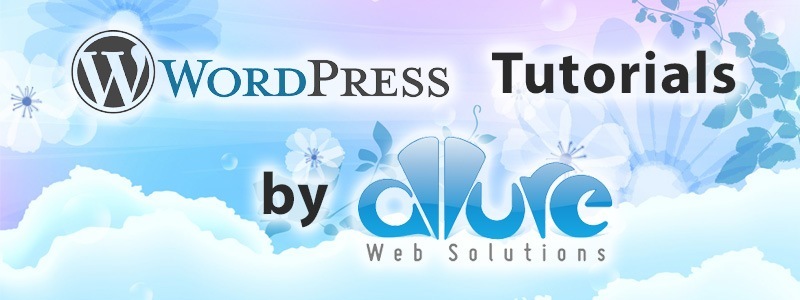
In this blog post, I will show how to make the meta slider have responsive images using the built in WordPress srcset
functionality. In order to do this we have two components. One is a function that gets a WordPress attachment’s ID from its URL. The other is the function that hooks into the meta slider and adds the srcset attribute.
Get Attachment ID from URL
This is a function I found online that performs a query on the database using an attachment’s URL to find it’s ID. Of course this isn’t 100% reliable if the link between the URL and the ID somehow breaks in the db, but it should work in almost all cases.
/** * Get an attachment ID given a URL. * * @param string $url * * @return int Attachment ID on success, 0 on failure */ function get_attachment_id_from_url( $attachment_url = '' ) { global $wpdb; $attachment_id = false; // If there is no url, return. if ( '' == $attachment_url ) { return; } // Get the upload directory paths $upload_dir_paths = wp_upload_dir(); // Make sure the upload path base directory exists in the attachment URL, to verify that we're working with a media library image if ( false !== strpos( $attachment_url, $upload_dir_paths['baseurl'] ) ) { // If this is the URL of an auto-generated thumbnail, get the URL of the original image $attachment_url = preg_replace( '/-\d+x\d+(?=\.(jpg|jpeg|png|gif)$)/i', '', $attachment_url ); // Remove the upload path base directory from the attachment URL $attachment_url = str_replace( $upload_dir_paths['baseurl'] . '/', '', $attachment_url ); // Finally, run a custom database query to get the attachment ID from the modified attachment URL $attachment_id = $wpdb->get_var( $wpdb->prepare( "SELECT wposts.ID FROM $wpdb->posts wposts, $wpdb->postmeta wpostmeta WHERE wposts.ID = wpostmeta.post_id AND wpostmeta.meta_key = '_wp_attached_file' AND wpostmeta.meta_value = '%s' AND wposts.post_type = 'attachment'", $attachment_url ) ); } return $attachment_id; } // Credits: https://philipnewcomer.net/2012/11/get-the-attachment-id-from-an-image-url-in-wordpress/
Hook into Meta Slider to Add SrcSet Attribute to Slides
There are a few things going on here. First we add a filter to specify the max srcset width available. By default, WordPress only goes up the “large” image size. Then we set the image attributes of each slider image with a function that pulls in the srcset values for that image from WordPress itself. Lastly, we unset the max srcset width so that it’s back to default and doesn’t affect the rest of the WordPress functionality.
/** * @param $image_attributes * @param $slide * @param $slider_id * * @return mixed */ function add_responsive_attributes_to_homepage_flex_slider_slides( $image_attributes, $slide, $slider_id ) { add_filter( 'max_srcset_image_width', 'max_srcset_slider', 10, 10 ); $image_attributes['srcset'] = wp_get_attachment_image_srcset( get_attachment_id_from_url( $slide['src'] ) ); $image_attributes['sizes'] = wp_get_attachment_image_sizes( get_attachment_id_from_url( $slide['src'] ), 'large' ); remove_filter( 'max_srcset_image_width', 'max_srcset_slider', 10 ); return $image_attributes; } function max_srcset_slider( $max_width, $size_array ) { return 1800; // or however big the largest image size can be } add_filter( 'metaslider_flex_slider_image_attributes', 'add_responsive_attributes_to_homepage_flex_slider_slides', 10, 10 );