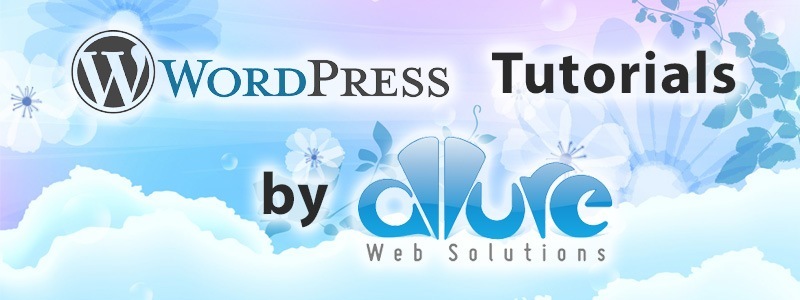
In the post, I show how to add javascript to your admin pages. This JS will only run when you are inside the WordPress dashboard. The example I use is for adding a custom meta box for a secondary featured image in the context of the Sage 9 theme.
The JS
Add this code to a new file called admin.js inside resources/assets/scripts/
import $ from 'jquery'; $(document).ready(function ($) { // Uploading files var file_frame; $.fn.upload_listing_image = function (button) { var button_id = button.attr('id'); var field_id = button_id.replace('_button', ''); // If the media frame already exists, reopen it. if (file_frame) { file_frame.open(); return; } // Create the media frame. file_frame = wp.media.frames.file_frame = wp.media({ title: $(this).data('uploader_title'), button: { text: $(this).data('uploader_button_text'), }, multiple: false, }); // When an image is selected, run a callback. file_frame.on('select', function () { var attachment = file_frame.state().get('selection').first().toJSON(); $("#" + field_id).val(attachment.id); $("#bannerimagediv img").attr('src', attachment.url); $('#bannerimagediv img').show(); $('#' + button_id).attr('id', 'remove_listing_image_button'); $('#remove_listing_image_button').text('Remove listing image'); }); // Finally, open the modal file_frame.open(); }; $('#bannerimagediv').on('click', '#upload_listing_image_button', function (event) { event.preventDefault(); $.fn.upload_listing_image($(this)); }); $('#bannerimagediv').on('click', '#remove_listing_image_button', function (event) { event.preventDefault(); $('#upload_listing_image').val(''); $('#bannerimagediv img').attr('src', ''); $('#bannerimagediv img').hide(); $(this).attr('id', 'upload_listing_image_button'); $('#upload_listing_image_button').text('Set listing image'); }); });
As part of this step, add the below to resources/assets/config.json
right below the customizer. This allows webpack to copy the admin.js file to the build folder.
"admin": [ "./scripts/admin.js" ]
The PHP
Add this to app/admin.php
below the customizer to enqueue our new admin.js file:
/** * Admin JS */ add_action('admin_enqueue_scripts', function () { wp_enqueue_script('sage/admin.js', asset_path('scripts/admin.js'), ['jquery'], null, true); });
Now that’s all your really need to have JS firing only inside the dashboard. But, as promised, below the example continues to show how to add a secondary featured image to posts and pages.
How To Add A Secondary Featured Image To Posts & Pages
This code can be added to app/helpers.php
or functions.php
if you’re using Sage 9. I also tend to put custom fields and meta boxes inside their own files (on the rare occasion I don’t use the ACF plugin).
/** * Add Secondary Featured Image Meta Box */ function secondary_featured_image_add_metabox() { $post_types = Array('post', 'page'); foreach ($post_types as $post_type) { add_meta_box('secondaryfeaturedimage', __('Secondary Featured Image', 'text-domain'), __NAMESPACE__ . '\\secondary_featured_image_metabox', $post_type, 'side', 'low'); } } add_action('add_meta_boxes', __NAMESPACE__ . '\\secondary_featured_image_add_metabox'); /** * * Secondary Featured Image Meta Box Content * * @param $post */ function secondary_featured_image_metabox($post) { global $content_width, $_wp_additional_image_sizes; $image_id = get_post_meta($post->ID, '_listing_image_id', true); $old_content_width = $content_width; $content_width = 254; if ($image_id && get_post($image_id)) { if (!isset($_wp_additional_image_sizes['post-thumbnail'])) { $thumbnail_html = wp_get_attachment_image($image_id, array($content_width, $content_width)); } else { $thumbnail_html = wp_get_attachment_image($image_id, 'post-thumbnail'); } if (!empty($thumbnail_html)) { $content = $thumbnail_html; $content .= '<p class="hide-if-no-js"><a href="javascript:;" id="remove_listing_image_button" >' . esc_html__('Remove secondary featured image', 'text-domain') . '</a></p>'; $content .= '<input type="hidden" id="upload_listing_image" name="_listing_cover_image" value="' . esc_attr($image_id) . '" />'; } $content_width = $old_content_width; } else { $content = '<img src="" style="width:' . esc_attr($content_width) . 'px;height:auto;border:0;display:none;" />'; $content .= '<p class="hide-if-no-js"><a title="' . esc_attr__('Set secondary featured image', 'text-domain') . '" href="javascript:;" id="upload_listing_image_button" id="set-listing-image" data-uploader_title="' . esc_attr__('Choose an image', 'text-domain') . '" data-uploader_button_text="' . esc_attr__('Set banner image', 'text-domain') . '">' . esc_html__('Set banner image', 'text-domain') . '</a></p>'; $content .= '<input type="hidden" id="upload_listing_image" name="_listing_cover_image" value="" />'; } echo $content; } /** * * Save Secondary Featured Image Meta Box * * @param $post_id */ function secondary_featured_image_meta_save($post_id) { if (isset($_POST['_listing_cover_image'])) { $image_id = (int)$_POST['_listing_cover_image']; update_post_meta($post_id, '_listing_image_id', $image_id); } } add_action('save_post', __NAMESPACE__ . '\\secondary_featured_image_meta_save', 10, 1);
Helper Function To Display Featured Image In Theme
/** * * Get Secondary Featured Image Meta * * @param $value * @return bool|string */ function secondary_featured_image_get_meta($value) { global $post; $image_id = get_post_meta($post->ID, $value, true); if (!empty ($image_id)) { return is_array($image_id) ? stripslashes_deep($image_id) : stripslashes(wp_kses_decode_entities($image_id)); } else { return false; } } /***** Usage _____ <?php echo wp_get_attachment_image_src( App\secondary_featured_image_get_meta('_listing_image_id'), 'full')[0]; ?> *****/