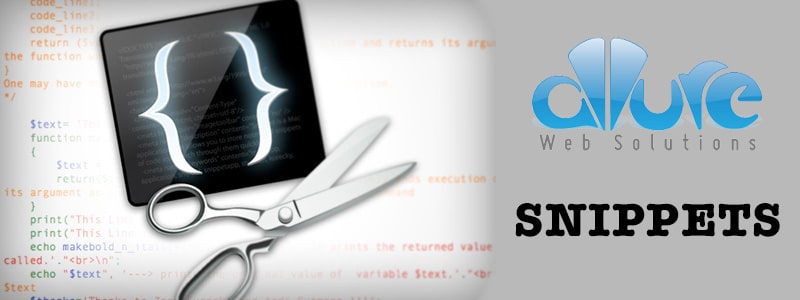
List of Mixins
- Lighten/Darken
- Placeholder styling
- PX to EMs
- PX to REMs with Fallback
- Vertical Alignment
- Breakpoints
- SVG Background Images with PNG and Retina Fallback
- Cross-Browser Compatible Opacity
Lighten / Darken
/********************* LIGHTEN / DARKEN *********************/ @function lighten($color, $percentage) { @return mix(white, $color, $percentage); } @function darken($color, $percentage) { @return mix(black, $color, $percentage); }
Placeholders
/********************* PLACEHOLDERS *********************/ @mixin optional-at-root($sel) { @at-root #{if(not &, $sel, selector-append(&, $sel))} { @content; } } @mixin placeholder { @include optional-at-root('::-webkit-input-placeholder') { @content; } @include optional-at-root(':-moz-placeholder') { @content; } @include optional-at-root('::-moz-placeholder') { @content; } @include optional-at-root(':-ms-input-placeholder') { @content; } } /**** USAGE .foo { @include placeholder { color: green; } } @include placeholder { color: red; } *****/
PX to EMs
/********************* PX to EMS *********************/ $browser-context: 16; // Default @function em($pixels, $context: $browser-context) { @return #{$pixels/$context}em; } /* USAGE */ font-size: em(16);
PX to REMs with Fallback
/********************* PX TO REMS *********************/ @function calculateRem($size) { $remSize: $size / 16px; @return $remSize * 1rem; } @mixin font-size($size) { font-size: $size; font-size: calculateRem($size); } /* USAGE */ p { @include font-size(14px) }
Vertical Alignment
/********************* VERTICAL ALIGNMENT *********************/ @mixin vertical-align($position: relative) { position: $position; top: 50%; -webkit-transform: translateY(-50%); -ms-transform: translateY(-50%); transform: translateY(-50%); } /* USAGE */ @include vertical-align(); // on the parent element you can add the following to remove any blurriness<code class=" language-css"> transform-style: preserve-3d;
Breakpoints
Credits to CSS-Tricks
/********************* BREAKPOINTS *********************/ $MQs: true; @mixin bp($point) { @if ($MQs) { $bp-small: "(max-width: 600px)"; $bp-medium: "(max-width: 1250px)"; $bp-large: "(max-width: 1600px)"; @if $point == large { @media #{$bp-large} { @content; } } @else if $point == medium { @media #{$bp-medium} { @content; } } @else if $point == small { @media #{$bp-small} { @content; } } } } /* USAGE */ .sidebar { width: 33.33%; @include bp(small) { width: 100%; } }
So how do you create both a style.css and a style-NoMQs.css from the same source Sass? We use the power of our @mixin.
We use a logic condition within the bp @mixin which will either continue and output the media query, or output nothing.
You wouldn’t declare that variable right outside the @mixin though. You would declare it from your “master” file that @imports all the partials. So perhaps your style.scss file is:
$MQs: true; @import "variables"; @import "colors"; @import "global"; /* etc. */
And you create the “No Media Queries” version (style-NoMQs.css) by creating a style-NoMQs.scss:
$MQs: false; @import "variables"; @import "colors"; @import "global"; /* etc. */
Just change that variable to false, and the output CSS will contain no media queries at all.
SVG background images with PNG and retina fallback
Credits to zerosixthree
This mixin relies on Modernizr. The .svg file will serve as the default background image. Next a .png serves as a fallback for non-svg-supporting browsers. Finally, you will add a .png that is 2x the size as a secondary fallback for retina screens.
All in all you need this:
- pattern.svg
- pattern.png
- pattern@2x.png
/************************************************* SVG BACKGROUND IMAGES WITH PNG AND RETINA FALLBACK **************************************************/ $image-path: '../img' !default; $fallback-extension: 'png' !default; $retina-suffix: '@2x'; @mixin background-image($name, $size:false){ background-image: url(#{$image-path}/#{$name}.svg); @if($size){ background-size: $size; } .no-svg &{ background-image: url(#{$image-path}/#{$name}.#{$fallback-extension}); @media only screen and (-moz-min-device-pixel-ratio: 1.5), only screen and (-o-min-device-pixel-ratio: 3/2), only screen and (-webkit-min-device-pixel-ratio: 1.5), only screen and (min-device-pixel-ratio: 1.5) { background-image: url(#{$image-path}/#{$name}#{$retina-suffix}.#{$fallback-extension}); } } } /* USAGE */ body { @include background-image('pattern'); }
Cross-Browser Compatible Opacity
With this mixin cross-browser compatibility for the opacity attribute will go all the way down to IE5.
/******************** CROSS BROWSER OPACITY *********************/ @mixin opacity($opacity) { opacity: $opacity; $opacity-ie: $opacity * 100; filter: alpha(opacity=$opacity-ie); //IE8 } /* USAGE */ .faded-text { @include opacity(0.8); }