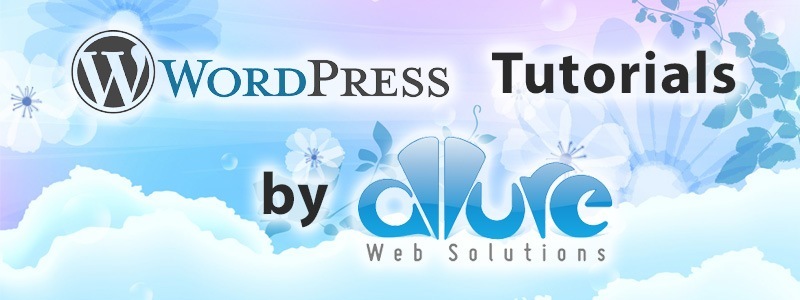
Here are several WordPress tips and tricks for when you are developing your own custom plugins. All of these make for a pretty good user experience. This page will be updated as I get new ideas!
- Add Settings Link
- Set Default Settings on Plugin Activation
- Redirect to Settings Page on Activation
- Create Admin Notice
Add Settings Link
/** * Add settings link to plugin page * * @param $links * @return mixed */ public function add_settings_link($links) { $settings_link = '<a href="options-general.php?page=wp-post-modal">' . __('Settings') . '</a>'; array_push($links, $settings_link); return $links; } add_filter('plugin_action_links_' . plugin_basename( __FILE__ ), 'add_settings_link');
If you are using the WordPress Plugin Boilerplate, your add_filter()
function would look like: $this->loader->add_filter('plugin_action_links_' . plugin_basename(plugin_dir_path(__DIR__) . $this->plugin_name . '.php'), $plugin_admin, 'add_settings_link');
Set Default Settings on Plugin Activation
This is an example for setting a checkbox to be checked by default.
Assuming code for rendering checkbox looks something like this: <input type="checkbox" name="checkbox" id="checkbox" value="1" <?php echo checked($styling, '1'); ?> />
register_activation_hook( __FILE__, 'myplugin_plugin_activate' ); function myplugin_plugin_activate() { if (get_option('wp_post_modal_styling') === false || get_option('wp_post_modal_styling') === '') update_option('wp_post_modal_styling', '1'); }
Replace OPTION_NAME with appropriate name of your option and on update_option()
update true
to your content for the option as needed.
Redirect to Settings Page on Activation
register_activation_hook( __FILE__, 'myplugin_plugin_activate' ); function myplugin_plugin_activate() { // redirect to settings page after plugin activation global $pagenow; if (isset($_GET['activated']) && $pagenow == 'plugins.php') { wp_redirect(admin_url('options-general.php?page=PLUGIN_NAME')); exit; } }
Replace PLUGIN_NAME or the whole URL options-general.php?page=PLUGIN_NAME
with the appropriate URL of your settings page.
Create an Admin Notice
add_filter('admin_notices', 'admin_notice'); add_filter('network_admin_notices', 'admin_notice'); // also show message on multisite public function admin_notice() { $message = '<h4>Thanks for installing WP Post Popup!</h4>'; ?> <div class="notice notice-success is-dismissible"> <?php echo $message; ?> <button type="button" class="notice-dismiss"> <span class="screen-reader-text">Dismiss this notice.</span> </button> </div> <?php }